산술 연산
(주의) 기본적인 단위는 동일해야 함
다른 단위의 잘못된 만남
div {
width: 100% - 200px; //에러
}
대신 calc() 칼크 함수로 다른 단위의 연산도 가능
div {
width: calc(100% - 200px);
}
SCSS
div {
width: 20px + 20px;
height: 40px - 10px;
font-size: 10px * 2;
margin: 30px / 2; // 주의
padding: 20px % 7; // 나머지
}
CSS
div {
width: 40px;
height: 30px;
font-size: 20px;
margin: 30px/2; /*단축속성 슬래시네?*/
padding: 6px;
}
나누기 연산자 - 세 가지 방법
나누기 연산자로
/
슬래시를 그냥 사용 못하는 이유
→ 단축 속성으로 해석될 여지가 있음단축 속성 font
/
슬래시로 구분font: font-size / line-height font-family;
기본적으로
font-family
도 명시해줘야해서 생각보다 유용하진 않음SCSS
span { font-size: 10px; line-height: 10px; font-family: serif; font: 10px / 10px serif; }
CSS
span { font-size: 10px; line-height: 10px; font-family: serif; font: 10px/10px serif; }
(첫번째 방법) 나누기 연산할 부분 ( )
소괄호로 묶어주기
SCSS
div {
margin: (30px / 2);
}
CSS
div {
margin: 15px;
}
(두번째 방법) 변수에 나누기 적용하기
SCSS
div {
$size: 30px;
margin: $size / 2;
}
CSS
div {
margin: 15px;
}
(세번째 방법) 다른 연산자와 함께 사용
- 곱하기나 나누기가 더하기나 빼기보다 우선하는 거 잊지 말기
SCSS
div {
margin: 10px + 12px / 2;
// -> 10 + 6
padding: (10px + 12px) / 2;
// -> 22 / 2
}
CSS
div {
margin: 16px;
padding: 11px;
}
Mixins
코드의 재활용. 아주 유용함
변수랑 비슷한데, 변수와는 다르게 여러가지 스타일을 한번에 담아 넣을 수 있음
기본 예제
@mixin으로 설정
@include로 가져오기
아래 예시에서는 center라는 이름으로 재활용 될 수 있음
SCSS
@mixin center {
display: flex;
justify-content: center;
align-items: center;
}
.container {
@include center;
.item {
@include center;
}
}
.box {
@include center;
}
CSS
.container {
display: flex;
justify-content: center;
align-items: center;
}
.container .item {
display: flex;
justify-content: center;
align-items: center;
}
.box {
display: flex;
justify-content: center;
align-items: center;
}
인수
JS의 함수를 선언하고 실행하는 부분과 유사함
SCSS
@mixin box($size) { // 매개변수
width: $size;
height: $size;
background-color: tomato;
}
.container {
@include box(200px); // 인수
.item {
@include box(100px);
}
}
.box {
@include box(100px);
}
CSS
.container {
width: 200px;
height: 200px;
background-color: tomato;
}
.container .item {
width: 100px;
height: 100px;
background-color: tomato;
}
.box {
width: 100px;
height: 100px;
background-color: tomato;
}
매개변수에 기본값을 지정하는 방법
매개변수에 미리 기본값을 정해두면 따로 인수가 없을 때는 지정해놨던 기본값을 적용
SCSS
@mixin box($size: 100px) {
width: $size;
height: $size;
background-color: tomato;
}
.container {
@include box(200px);
.item {
@include box;
}
}
.box {
@include box;
}
CSS
.container {
width: 200px;
height: 200px;
background-color: tomato;
}
.container .item {
width: 100px;
height: 100px;
background-color: tomato;
}
.box {
width: 100px;
height: 100px;
background-color: tomato;
}
여러 개의 매개변수
쉼표로 구분해요
SCSS
@mixin box($size: 100px, $color: tomato) {
width: $size;
height: $size;
background-color: $color;
}
.container {
@include box(200px, blue);
.item {
@include box;
}
}
.box {
@include box;
}
CSS
.container {
width: 200px;
height: 200px;
background-color: blue;
}
.container .item {
width: 100px;
height: 100px;
background-color: tomato;
}
.box {
width: 100px;
height: 100px;
background-color: tomato;
}
키워드 인수
원래 순서대로 인수를 넣어줘야 하는데, 기본값과 동일한 인수는 굳이 적기 싫단 말이지.
인수의 순서와 상관 없이 값을 지정하고 싶은 인수의 키워드를(매개변수명) 입력하고 값을 주면 끝!
SCSS
@mixin box($size: 100px, $color: tomato) {
width: $size;
height: $size;
background-color: $color;
}
.container {
@include box(200px, blue);
.item {
@include box(100px, red);
//사이즈 기본값인데 굳이 적어야함?
}
}
.box {
@include box($color: green);
//키워드 인수 사용하면 해결됨
}
CSS
.container {
width: 200px;
height: 200px;
background-color: blue;
}
.container .item {
width: 100px;
height: 100px;
background-color: red;
}
.box {
width: 100px;
height: 100px;
background-color: green;
}
반복문
어디서 유용하게 쓰이나?
→ 오버워치 애들 사진 넣었던 거 기억남? ㅇㅇ...
참고) JS의 반복문
for (let i = 0; i < 10; i += 1) { console.log(`loop-${i}`) }
제로 베이스드가 아님
@for $i from 1 through 10 { //제로 베이스드 아님
.box {
width: 100px;
}
}
.box {
width: 100px;
}
/*이거 10개 나옴*/
보간법
기호 주의하기
#
보간법 왜 쓰나?
.box:nth-child()
얘는 하나의 CSS 선택자선택자에서는 i라는 변수가 가지고 있는 숫자를 그대로 명시할 수 없음
그래서 문자를 보간하는 방식을 써야 함
@for $i from 1 through 10 {
.box:nth-child(#{$i}) { //SCSS식 보간법
width: 100px;
}
}
.box:nth-child(1) {
width: 100px;
}
.box:nth-child(2) {
width: 100px;
}
/* 생략 */
.box:nth-child(10) {
width: 100px;
}
실제 값을 적는 부분은 보간할 필요 없음
실제로 값을 적는 부분이어서 보간 해줄 필요 없음
(값을 명시하는 부분. i라는 변수를 실제로 사용하면 되는.)
선택자 같은 경우에는 값을 적는 부분이 아니어서 보간 처리를 한 거라고 보면 됨
@for $i from 1 through 10 {
.box:nth-child(#{$i}) {
width: 100px * $i;
}
}
.box:nth-child(1) {
width: 100px;
}
.box:nth-child(2) {
width: 200px;
}
/* 생략 */
.box:nth-child(10) {
width: 1000px;
}
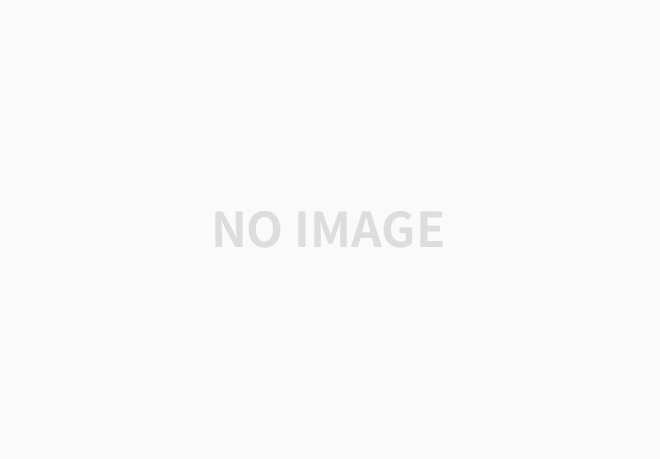
날아간 줄 알았던 CSS 자료를 찾았다
흑흑 이제 모르는 거 나와도 덜 두려워
https://bit.ly/37BpXiC
본 포스팅은 패스트캠퍼스 환급 챌린지 참여를 위해 작성되었습니다.
#직장인인강 #직장인자기계발 #패스트캠퍼스 #패스트캠퍼스후기 #패캠챌린지 #한번에끝내는프론트엔드개발초격차패키지Online
'패스트캠퍼스' 카테고리의 다른 글
Bundler [패스트캠퍼스 챌린지 22일차] (0) | 2021.09.27 |
---|---|
SCSS Sass 마지막!! [패스트캠퍼스 챌린지 21일차] (0) | 2021.09.26 |
SCSS Sass 1 [패스트캠퍼스 챌린지 19일차] (0) | 2021.09.24 |
작성자와 사용자의 관점으로 코드 바라보기 [패스트캠퍼스 챌린지 18일차] (0) | 2021.09.23 |
타입스크립트 타입 4 [패스트캠퍼스 챌린지 17일차] (0) | 2021.09.22 |